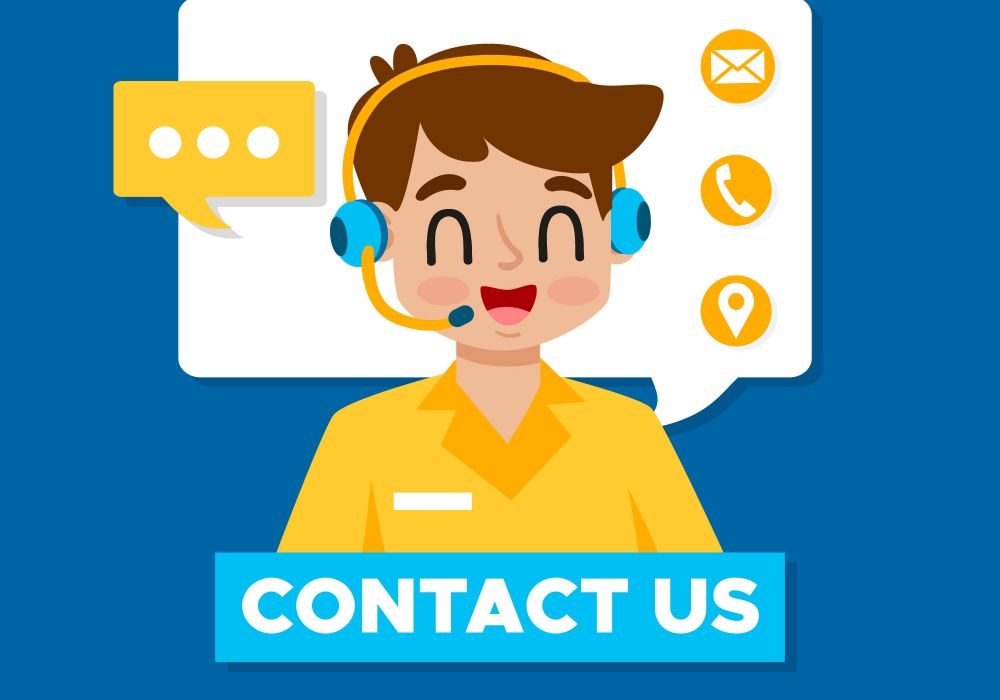
Using Your Gmail Account as SMTP Mail Server to Send Contact Us in Laravel
Send Email in Laravel for Contact Us Form
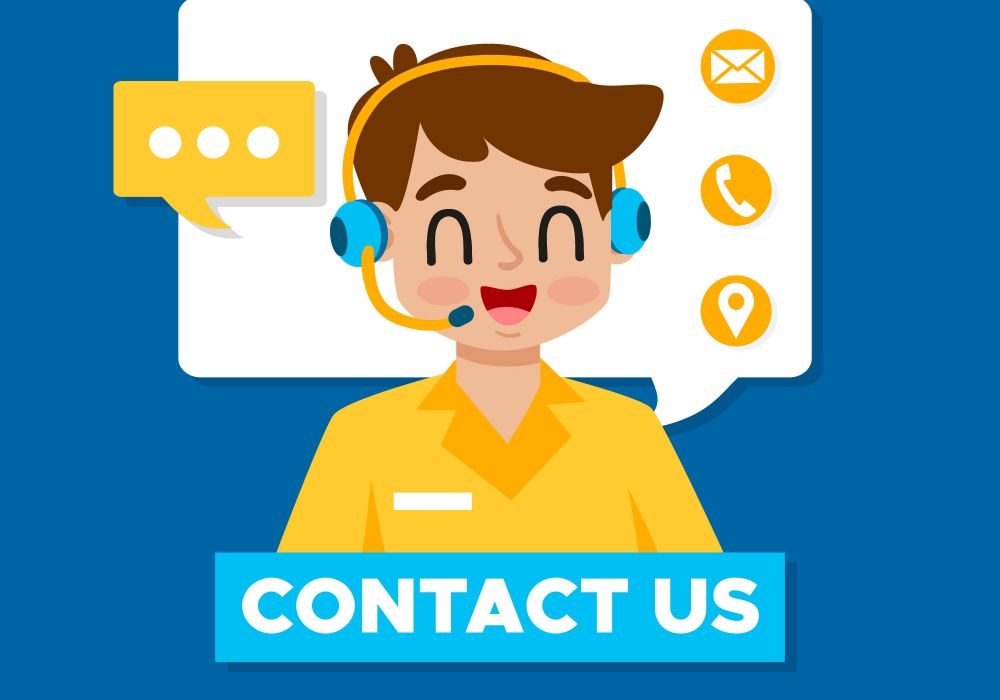
As you already know most websites always have a contact us form, where visitors can communicate with blogs or website owners based on their needs. Configuring or finding a mail server is time-consuming, costing, difficult when all you want is just the credential of an SMTP mail server. Gmail is one of the most popular email services for users to receive email from someone who knows their email address. Do You Know Your Gmail Account can Act as Your STMP Mail Server for Out-Going Sending Email? In this post, I am going to create a small Laravel program by using a Gmail account as an SMTP mail server to send the contact us form.
Prerequisite: If you want to try this example, you have to have basic knowledge of HTML, Bootstrap (For design contact us form) Laravel (Route, Controller, Model, Trait), and some of the command to run your laravel application.
Step 1: Create a new route to show the contact us form
- Create route /contact-us in folder routes/web.php
Route::get('/contact-us', 'IndexController@getContactUs')->name('contactus');
- Create a new controller IndexController and write a function
public function getContactUs(){
$title = "Contact Us";
return view('pages.contact-us', compact('title'));
}
- Create a new view in the folder views/pages/contact-us.blade.php
Step 2: Using HTML and Boostrap to design contact us form
You can freely design your contact us form based on your skill of HTML and bootstrap, below is what I've done mine
<div class="page-title lb single-wrapper">
<div class="container">
<div class="row">
<div class="col-lg-8 col-md-8 col-sm-12 col-xs-12">
<h2><i class="fa fa-envelope-open-o bg-orange"></i> Contact us <small class="hidden-xs-down hidden-sm-down">Feel free to keep in touch and feedback us.</small></h2>
</div><!-- end col -->
<div class="col-lg-4 col-md-4 col-sm-12 hidden-xs-down hidden-sm-down">
<ol class="breadcrumb">
<li class="breadcrumb-item"><a href="{{url('/')}}">Home</a></li>
<li class="breadcrumb-item active">Contact</li>
</ol>
</div><!-- end col -->
</div><!-- end row -->
</div><!-- end container -->
</div><!-- end page-title -->
<section class="section wb">
<div class="container">
<div class="row">
<div class="col-lg-12">
<div class="page-wrapper">
<div class="row">
<div class="col-lg-5">
<h4>Who we are</h4>
<p>Nest Code is a knowledge hub of technology, coding tips, and a valuable platform for IT skill sharing.</p>
<h4>How we help?</h4>
<p>We can help to share your knowledge experiences, your coding tips, and providing consultancy on your future big IT projects. We also provide / develop solution based on your needs as well.</p>
<h4>Question</h4>
<p>If you are curious about us and wanted to know us more, please feel free to contact us<br/> Email: nestcode168@gmail.com<br/> Tel: (+855) 69 623 652</p>
</div>
<div class="col-lg-7">
@if (session('status_contactus'))
<div class="alert alert-success">
{!! session('status_contactus') !!}
</div>
@endif
<form class="form-wrapper" method="POST" action="{{ route('contact-us') }}">
@csrf
<input type="text" class="form-control" name="name" placeholder="Your name *" required>
<input type="email" class="form-control" name="email" placeholder="Email address *" required>
<input type="text" class="form-control" name="phone" placeholder="Phone *" required>
<input type="text" class="form-control" name="subject" placeholder="Subject *" required>
<textarea class="form-control" name="message" placeholder="Your message *" required></textarea>
<button type="submit" class="btn btn-primary">Send <i class="fa fa-envelope-open-o"></i></button>
</form>
</div>
</div>
</div><!-- end page-wrapper -->
</div><!-- end col -->
</div><!-- end row -->
</div><!-- end container -->
</section>
Then, you run PHP artisan serve and type 127.0.0.1:8000/contact-us to view your form
php aritsan serve
http://127.0.0.1:80000/contact-us
Step 3: Sending an Email
- Create a new post route to handle sending functionality when visitor click on send button after filling in all the information
Route::post('/contact-us', 'IndexController@saveContactUs')->name('contact-us');
- Write function saveContactUs in controller IndexController
?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Traits\Nestcode;
class IndexController extends Controller
{
use Nestcode;
public function getContactUs(){
$title = "Contact Us";
return view('pages.contact-us', compact('title'));
}
public function saveContactUs(Request $request){
$data = [
"name" => $request->get('name'),
"email" => $request->get('email'),
"phone" => $request->get('phone'),
"subject" => $request->get('subject'),
"message" => $request->get('message')
];
$this->sendEmail(['to' => [env('superadmemail')], 'data' => $data,
'subject' => 'You have got a new contact us message', 'attachments' =>[]]);
return redirect()->back()->with('status_contactus', 'Your messages has been successfully sent. Thank You!');
}
}
- Create a new Laravel Trait to code function sending email App/Traits/NestCode.php
<?php
namespace App\Traits;
use DB;
use Session;
use Carbon\Carbon;
use Cache;
trait Nestcode {
private function getSetting($name)
{
if (Cache::has('setting_'.$name)) {
return Cache::get('setting_'.$name);
}
$content = env($name);
Cache::forever('setting_'.$name, $content);
return $content;
}
public function sendEmail($config = [])
{
\Config::set('mail.driver', $this->getSetting('smtp_driver'));
\Config::set('mail.host', $this->getSetting('smtp_host'));
\Config::set('mail.port', $this->getSetting('smtp_port'));
\Config::set('mail.username', $this->getSetting('smtp_username'));
\Config::set('mail.password', $this->getSetting('smtp_password'));
$to = $config['to'];
$data = $config['data'];
$subject = $config['subject'];
$attachments = ($config['attachments']) ?: [];
\Mail::send("pages.email-blank", ['data' => $data], function ($message) use ($to, $subject, $attachments) {
$message->priority(1);
$message->to($to);
$from_name = $this->getSetting('appname');
$message->from($this->getSetting('email_sender'), $from_name);
if (count($attachments)) {
foreach ($attachments as $attachment) {
$message->attach($attachment);
}
}
$message->subject($subject);
});
}
}
- Configure Credential of Gmail Account as SMPT Mail Server in .env file to let the function sendEmail working
superadmemail = website_owner@gmail.com //blog or website owner
appname = NestCode
email_sender = no-reply@nestcode.co //display name of email sender, you can put anything you like
smtp_driver = smtp
smtp_host = smtp.gmail.com
smtp_port = 587
smtp_username = your_gmail_account@gmail.com //Your gmail account
smtp_password = password_of_gmail //Your gmail password
- Design email content for delivery to blog or website owner views/pages/email-blank.blade.php
<section class="section wb">
<div class="container">
<div class="row">
<div class="col-lg-12">
<div class="page-wrapper">
<div class="row">
<div class="col-lg-5">
<h4>Subject: {!!$data['subject']!!}</h4>
<p>{!!$data['message']!!}</p>
</div>
<div class="col-lg-7">
<h4>Sender</h4>
<form class="form-wrapper">
<label>{!!$data['name']!!}</label> /
<label>{!!$data['email']!!}</label> /
<label>{!!$data['phone']!!}</label>
</form>
</div>
</div>
</div>
</div>
</div>
</div>
</section>
- Run command php artisan serve again, fill in the contact us information and click button send and website owner will receive an email in address website_owner@gmail.com