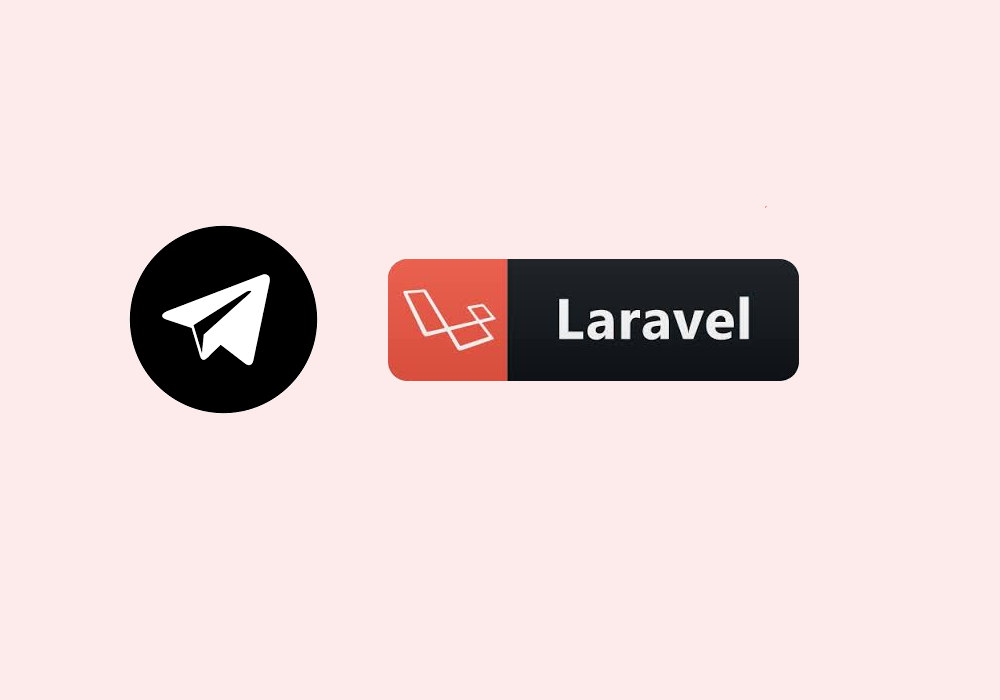
Using Telegram Bot to Share Information to Telegram Channel
Experiment on Laravel 7 by Sharing Article Posts
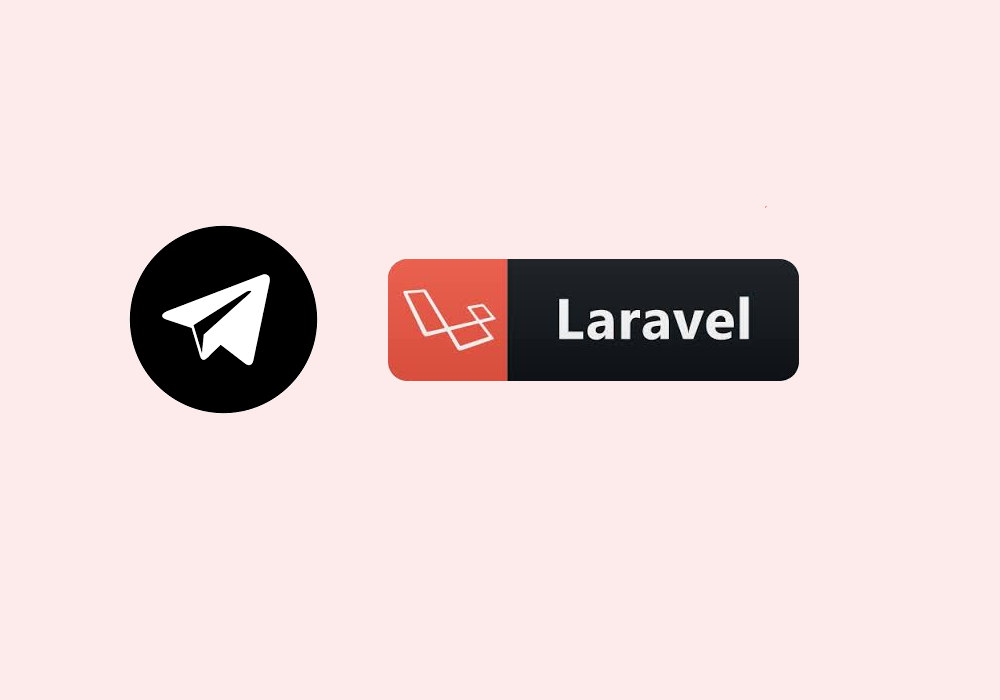
Telegram channel is another popular platform to connect with audiences which posts are controlled by channel owners. The Channel owner is the one responsible for providing knowledge, articles, and vital information for their subscribers. The subscriber needs to subscribe to the channel to receive that information. Normally, channel owners will write or copy/paste their content to the telegram channel for sharing, but in this post, we are going to introduce you to another way of sharing information using the telegram channel with coding to automate the process. It means that you don't have to copy/paste content anymore.
Using Telegram Bot as a robot or messager between your Content Management System (CMS) and your owned telegram channel will provide you some benefits such as time save for posting/sharing and one-click sharing from your CMS platform.
Step 1: Create Telegram Bot
- Let start by talking to BotFather. You can chat to @BotFather in the telegram app by sending command /start
- To create a new bot send command /newbot then it will be asked for a new bot's name
- Enter a username of your bot which needed to have prefix bot
- Finally, we will get an access token for your newly created bot
Step 2: Add this newly created bot as administrator on your telegram channel
Step 3: Installing Telegram Bot SDK to work with Laravel 7
composer require irazasyed/telegram-bot-sdk
- Add the following code snippet into providers array in config/app.php
Telegram\Bot\Laravel\TelegramServiceProvider::class,
- Add the following code snippet into alias array in config/app.php
'Telegram' => Telegram\Bot\Laravel\Facades\Telegram::class,
- Publish configuration of the Telegram Bot SDK to get telegram.php file in config folder
php artisan vendor:publish --provider="Telegram\Bot\Laravel\TelegramServiceProvider"
Copied File [\vendor\irazasyed\telegram-bot-sdk\src\Laravel\config\telegram.php] To [\config\telegram.php]
Publishing complete.
- Configure credential getting from BotFather to env file
TELEGRAM_BOT_NAME=your_bot_username
TELEGRAM_BOT_TOKEN=access_token_getting_from_bot_father
TELEGRAM_CERTIFICATE_PATH=
TELEGRAM_WEBHOOK_URL=
Step 4: Getting Channel CHAT_ID by coding since we can't get it from the telegram app. this CHAT_ID will be used in the further processes
- Create a controller TelegramBotController
php artisan make:controller TelegramBotController
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Telegram\Bot\Laravel\Facades\Telegram;
class TelegramBotController extends Controller
{
//
public function getChatID(){
$activity = Telegram::getUpdates();
dd($activity);
}
}
- Create a route to point to the controller
Route::get('/telegram_chatid', 'TelegramBotController@getChatID');
- Getting CHAT_ID
Then, add this CHAT_ID to the env file too
TELEGRAM_CHANNEL_ID=your_chat_id
Step 5: Sending your messages to Telegram Channel as needed with the following code
public function storeMessage(Request $request)
{
$request->validate([
'email' => 'required|email',
'message' => 'required'
]);
$text = "A new contact us query\n"
. "<b>Email Address: </b>\n"
. "$request->email\n"
. "<b>Message: </b>\n"
. $request->message;
Telegram::sendMessage([
'chat_id' => env('TELEGRAM_CHANNEL_ID', ''),
'parse_mode' => 'HTML',
'text' => $text
]);
return redirect()->back();
}
Here is the result example of Channel NestCode, you can join by click here https://t.me/nestcode168
As a result, whenever the posts are ready to be published to the channel all we have to do is clicking the button to post to the telegram channel.