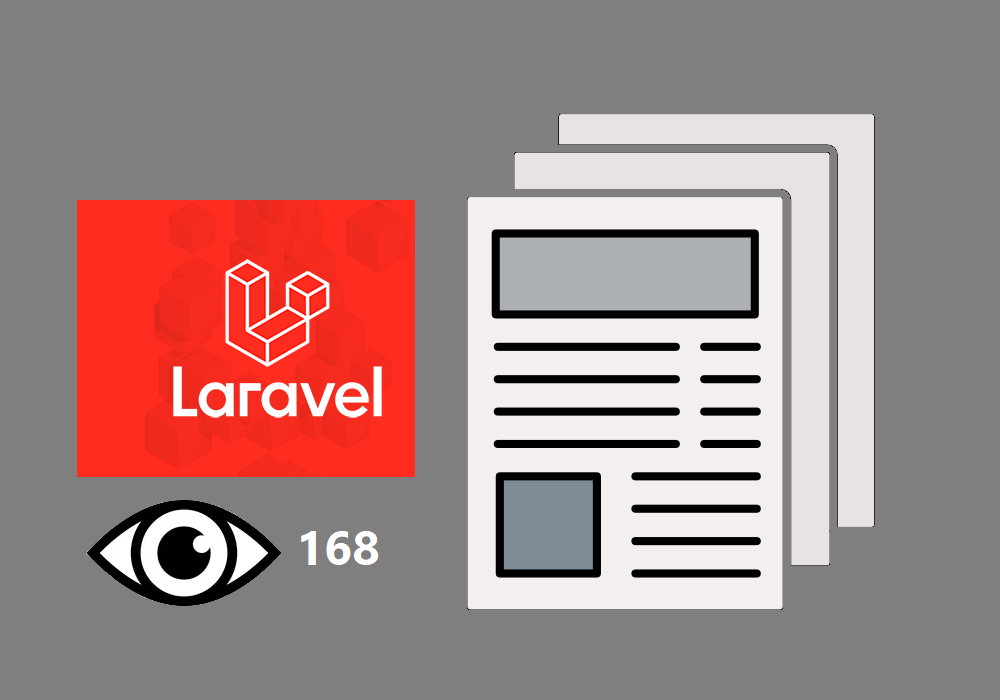
Tip to count view of an article in Laravel
Finding number of people viewing your posts
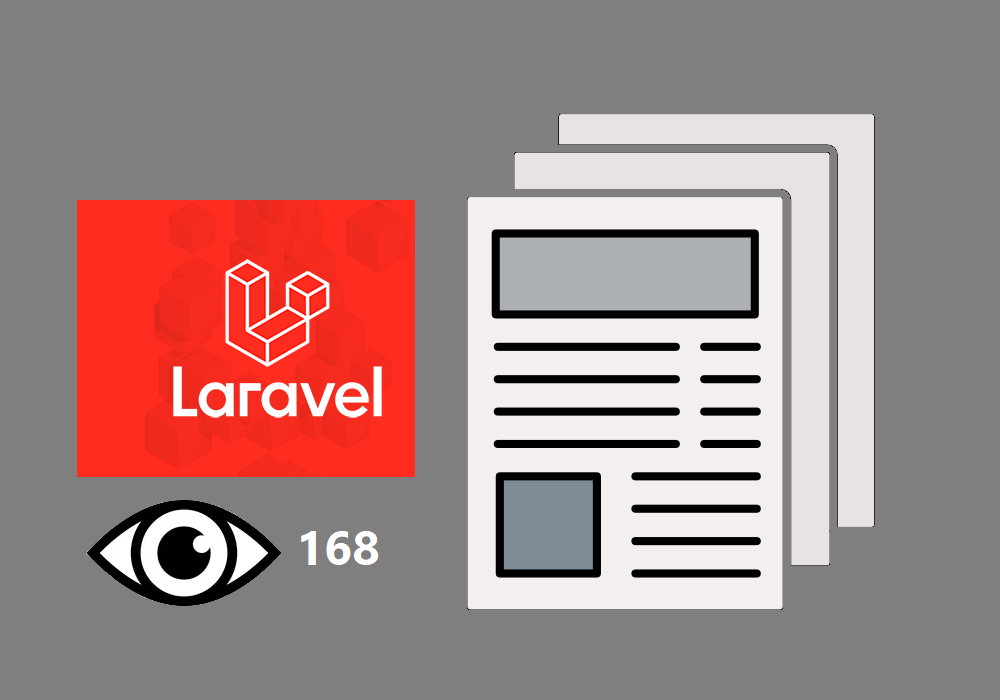
In this post, I would like to write a small coding tip on how you can count the number of views on an article or post in the laravel application. There are some benefits of page views. It allows you to track how web traffic interacts with your site and it filters out page refreshes and multiple page views in a single session. Yep, that's correct. the key here is using the session to count.
As usual, the first step you will do is to create a route to handle the request of viewing an article/post. Next, creating a controller to handle the task of getting article/post data from the database and send it to blade view to render. Finally, creating a blade view to design your article page using HTML and some special syntax of blade view.
Route::get('/{blogPostSlug}','BlogReaderController@viewSinglePost')->name('blog.single');
public function viewSinglePost(Request $request, $blogPostSlug)
{
// the published_at + is_published are handled by BinshopsBlogPublishedScope, and don't take effect if the logged in user can manage log posts
$blog_post = PostModel::where([
["slug", "=", $blogPostSlug]
])->firstOrFail();
return view("binshopsblog::single_post", [
'post' => $blog_post
]);
}
To count the number of views when each visitor clicks on the article, I will use the laravel trait to define a function of counting. A laravel trait is designed to the limitation of single inheritance by guiding developers to reuse sets of methods/functions freely in individual classes by staying in different class hierarchies.
- Create a trait class
<?php
namespace App\Traits;
use DB;
use Session;
trait Nestcode {
public function countView($slug){
$Key = 'key_' . $slug;
if (!Session::has($Key)) {
//increment view count by one
DB::table('binshops_posts')->join('binshops_post_translations', 'binshops_posts.id', 'binshops_post_translations.post_id')
->where('slug', $slug)->increment('view_count', 1);
Session::put($Key, 1);
}
}
}
- Include trait class and calling function in the controller which handles showing article/post
class BlogReaderController extends Controller
{
use Nestcode;
public function viewSinglePost(Request $request, $blogPostSlug)
{
$this->countView($blogPostSlug);
// the published_at + is_published are handled by BinshopsBlogPublishedScope, and don't take effect if the logged in user can manage log posts
$blog_post = PostModel::where([
["slug", "=", $blogPostSlug]
])->firstOrFail();
return view("binshopsblog::single_post", [
'post' => $blog_post
]);
}
}
The main focus of this post is about how you can use sessions to count the number of visitors who view your article/post.
THANK YOU!!!