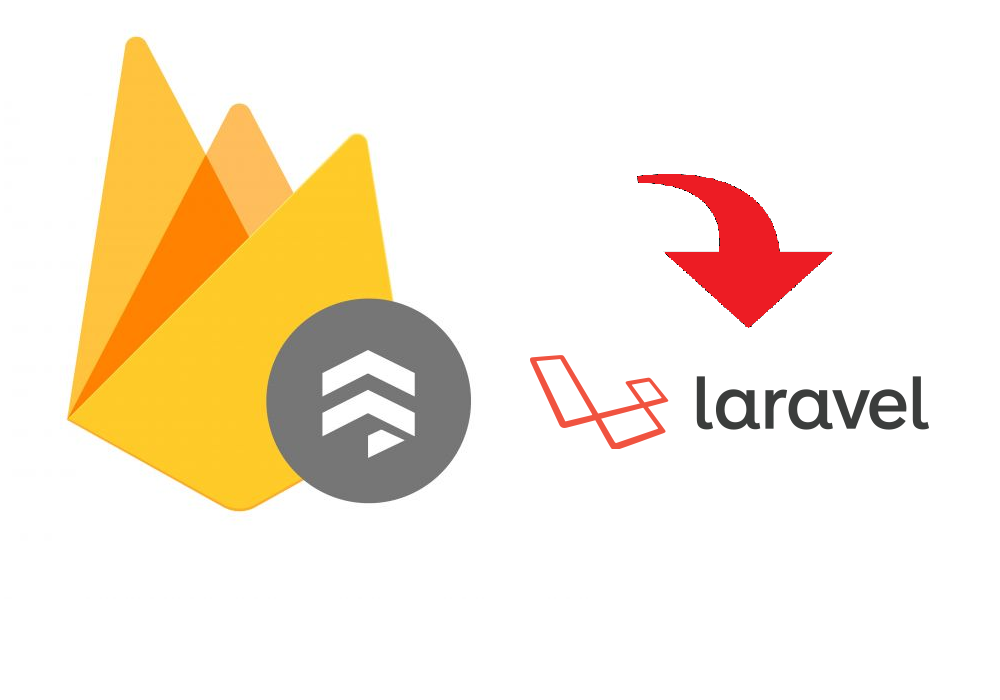
Fetching Data From Firebase Firestore Using Laravel
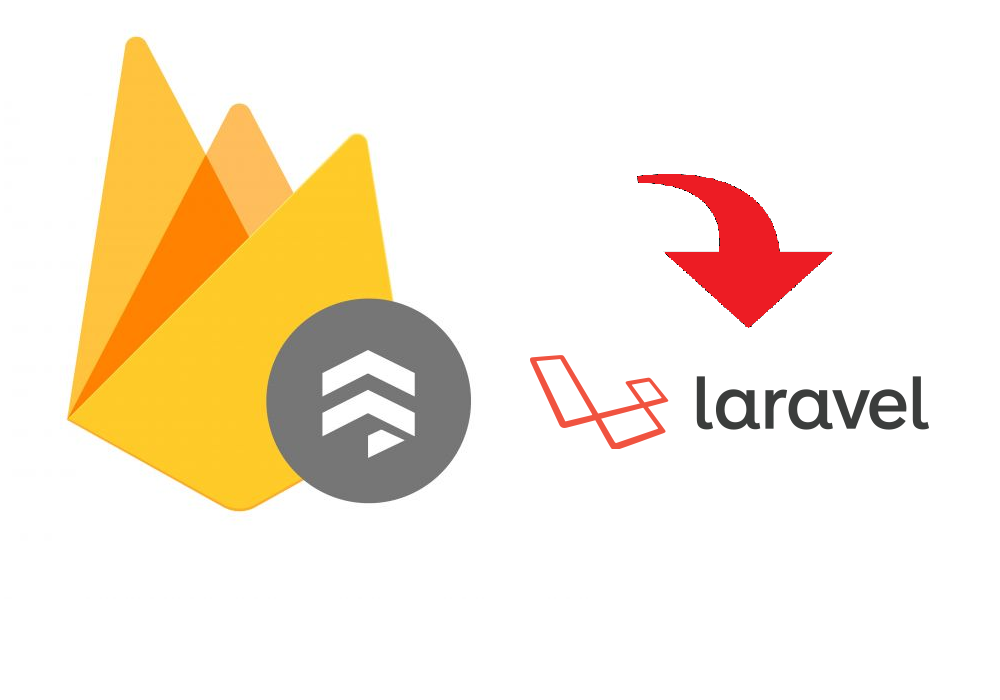
In this blog, we are going to introduce the know-how of fetching data from firebase firestore using laravel. This technique is inspired by the real project in which we are working with our client with the requirement of showing data that is stored in the firestore to administrators for patrol. Data stored in the firestore is the chatting/negotiating information of two people. Definition of firestore from the official website is a flexible, scalable database for mobile, web, and server development from Firebase and google cloud. Firestore is a cloud-hosted NoSQL database that enables iOS, Android, and web apps can access directly via native SDKs. Since firestore is a NoSQL database, therefore, data is stored as documents that contain fields mapping to values.
In our scenario, we have a mobile app that has features for users to chat and content is stored in firestore. This is done by using native SDKs provided by firebase. below is the schema of our model in firestore.
{
"room":[
{
"0ErM13WOc9iPrl0bzxTy":{
"chat_id":"xxxxx",
"created_by":"xxxxx",
"is_replied":"xxxxx",
"last_message":"xxxxx",
"last_message_id":"xxxxx",
"participant":[
"xxxxx",
"xxxxx"
],
"updated":"xxxxx",
"messages":[
{
"1a9b43QFcNvpGfIuyiZd":{
"content":"xxxxx",
"content_type":"xxxxx",
"is_read":"xxxxx",
"sender":"xxxxx",
"utc_timestamp":"xxxxx"
}
}
]
},
"2iJfoYCQQRs6IknY2suR":{
"chat_id":"xxxxx",
"created_by":"xxxxx",
"is_replied":"xxxxx",
"last_message":"xxxxx",
"last_message_id":"xxxxx",
"participant":[
"xxxxx",
"xxxxx"
],
"updated":"xxxxx",
"messages":[
{
"1a9b43QFcNvpGfIuyiZd":{
"content":"xxxxx",
"content_type":"xxxxx",
"is_read":"xxxxx",
"sender":"xxxxx",
"utc_timestamp":"xxxxx"
}
}
]
}
}
]
}
As you have seen, we have a bunch of array rooms that each room represented for pair conversation of 2 people which identified by a unique chat key. Each room has some information about participants and an array of messages that users communicate with each other. You may have different models of document storing in the firestore, but the concept is still the same.
Enabling Extensions
In order to connect and work with firebase firestore, we have to enable extensions "grpc" followed by the official document here. The configuration is varied by the operating system. Since we are working on OS windows and using the wamp server as a tool to run the apache webserver we have to download the DLL file of "grpc" extension from this website and add config to php.ini
Coding in Action
In the back-end, we use 3rd party laravel package ''firebase-php" to interact with Firebase by providing google service account credentials (JSON format) to get them ready to work with all services of Firebase. Using the following code to get all messages of conversation between 2 people (each conversation defined by a unique chat key)
//---- Create FireStore Client Object -----//
$serviceAccount = ServiceAccount::fromJsonFile(__DIR__ . '/API/' . env('GOOGLE_SERVICES_ACCOUNT'));
$factory = (new Factory)->withServiceAccount($serviceAccount);
$firestore = $factory->createFirestore();
$database = $firestore->database(); //FireStoreClient Object
$room = $database->collection('room');
$conversation = $room->document($chatkey); //$chatkey is parameter represent conversation of 2 people
$query = $conversation->collection('messages')->orderBy('utc_timestamp', 'desc');
$messages = $query->documents();
dd($messages);
Here is the result
THANK YOU