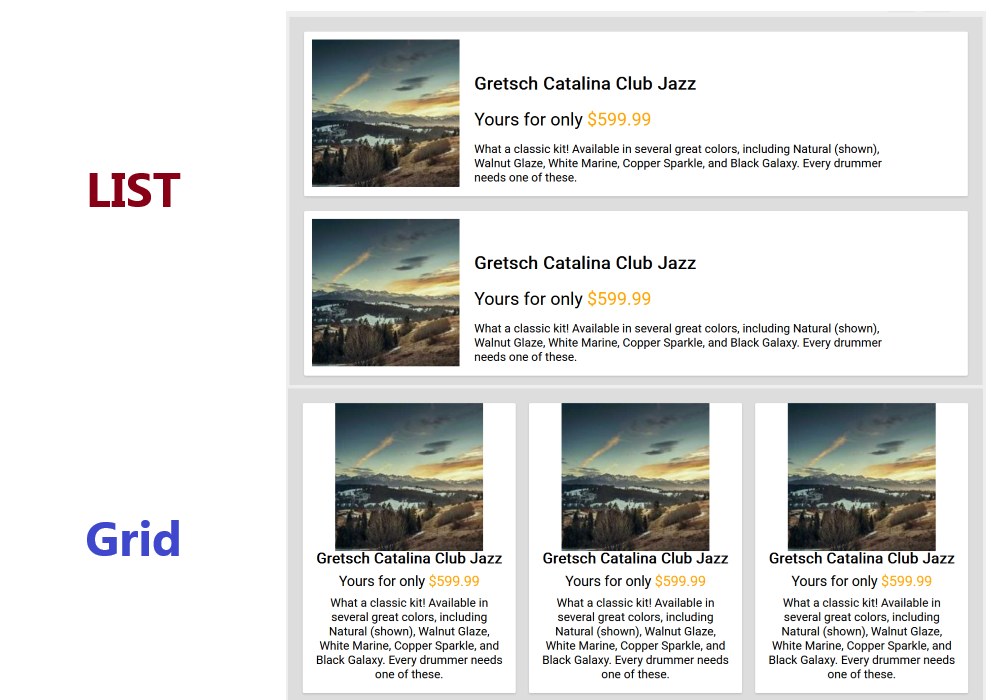
Toggle List and Grid View HTML
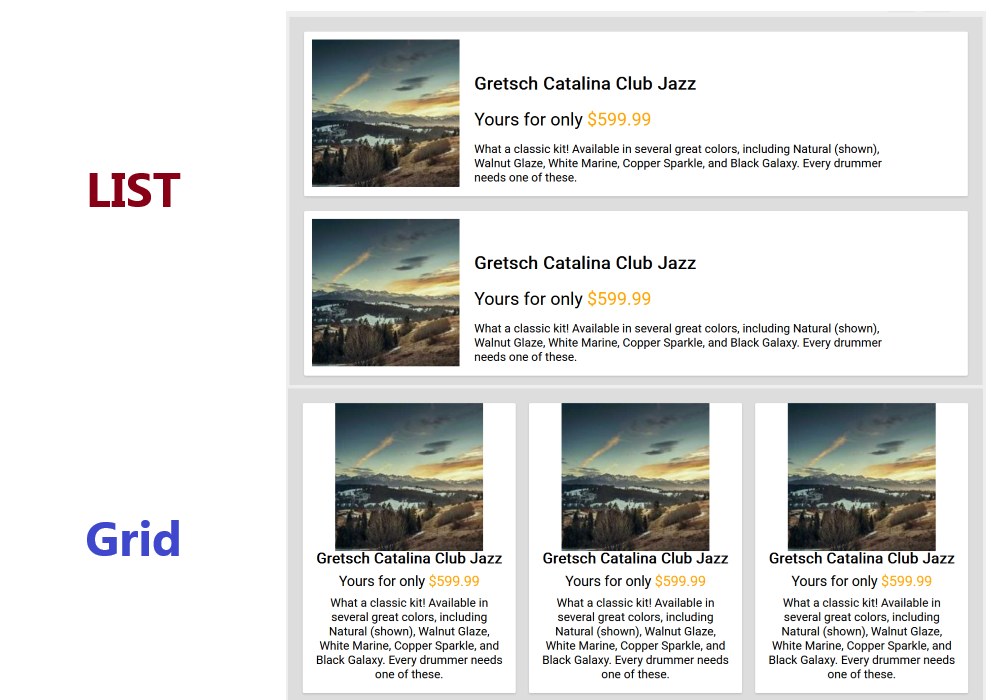
If you are a website designer you might come across a scenario where you want to display your items in two different modes List and Grid. These two modes of display are common in E-commerce websites for showing production names, short descriptions, models, or prices based on users' preferences. Some users have preferred list view mode and others prefer grid view mode. In this post, we will introduce a code snippet of how to design these two view modes using only JS, CSS, and HTML.
Below code is the design HTML script you can follow in no time
<div class="title">A Simple List/Grid Toggle</div>
<div class="buttons">
<button class="list-view on"><i class="fa fa-bars"></i></button>
<button class="grid-view"><i class="fa fa-th"></i></button>
</div>
<div class="wrapper list">
</div>
Stylesheet
@import url(https://fonts.googleapis.com/css?family=Roboto);
body {
background-color: #eee;
}
.title {
text-align: center;
position: absolute;
width: 100%;
font-size: 27px;
font-family: 'Roboto';
font-weight: 600;
z-index: -1;
}
.buttons {
max-width: 92%;
width: 900px;
text-align: right;
margin: 20px auto 0;
padding-right: 3%;
}
button {
height: 36px;
width: 40px;
margin-left: 4px;
font-size: 24px;
color: orange;
text-align: center;
line-height: 1.4;
border-radius: 4px;
border: none;
outline: none;
background-color: white;
box-shadow: 0px 1px 2px #bbb;
}
button:hover {
cursor: pointer;
box-shadow: 0px 0px 3px #666;
}
button.on {
color: white;
background-color: #ccc;
}
.wrapper {
max-width: 92%;
width: 900px;
margin: 10px auto 40px;
padding: 20px 20px 0;
background-color: #ddd;
font-family: Roboto;
position: relative;
overflow: hidden;
}
.item {
background-color: #fff;
overflow: hidden;
border-radius: 2px;
box-shadow: 0px 1px 3px #bbb;
}
img {
display: inline-block;
float: left;
max-height: 90%;
max-width: 90%;
padding: 1.2%;
}
a {
display: block;
transition: 0.2s;
}
a:hover {
opacity: 0.9;
cursor: pointer;
}
h2 {
transition: 0.2s;
}
h2:hover {
color: #666;
cursor: pointer;
}
span {
font-size: 24px;
}
span.price {
color: orange;
}
.list {
.item {
width: 100%;
margin: 0 auto 20px;
}
img {
max-width: 30%;
}
.details {
float: left;
max-width: 66%;
margin-top: 4%;
margin-left: 1%;
}
}
.grid {
.item {
width: 32%;
margin: 0 2% 20px 0;
float: left;
text-align: center;
}
.item:nth-child(3n) {
margin-right: 0;
}
img {
padding-top: 0;
max-width: 90%;
margin: 0 auto;
float: none;
}
h2 {
font-size: 20px;
margin: 10px 0;
}
span {
display: inline-block;
margin-top: -6px;
font-size: 19px;
}
.details {
float: none;
max-width: 90%;
margin: -20px auto 0;
p {
margin-top: 8px;
}
}
}
Javascript code for switching between list and grid view
howMany = 12;
listButton = $('button.list-view');
gridButton = $('button.grid-view');
wrapper = $('div.wrapper');
div = '<div class="item"><a href="javascript:void(0);"><img src="https://picsum.photos/200" /></a><div class="details"><h2>Gretsch Catalina Club Jazz</h2><span>Yours for only <span class="price">$599.99</span></span><p>What a classic kit! Available in several great colors, including Natural (shown), Walnut Glaze, White Marine, Copper Sparkle, and Black Galaxy. Every drummer needs one of these.</p></div></div>';
// Set up divs
for (i = 0; i < howMany; i++) {
$('.wrapper').append( div );
}
listButton.on('click',function(){
gridButton.removeClass('on');
listButton.addClass('on');
wrapper.removeClass('grid').addClass('list');
});
gridButton.on('click',function(){
listButton.removeClass('on');
gridButton.addClass('on');
wrapper.removeClass('list').addClass('grid');
});
DEMO in Codepen