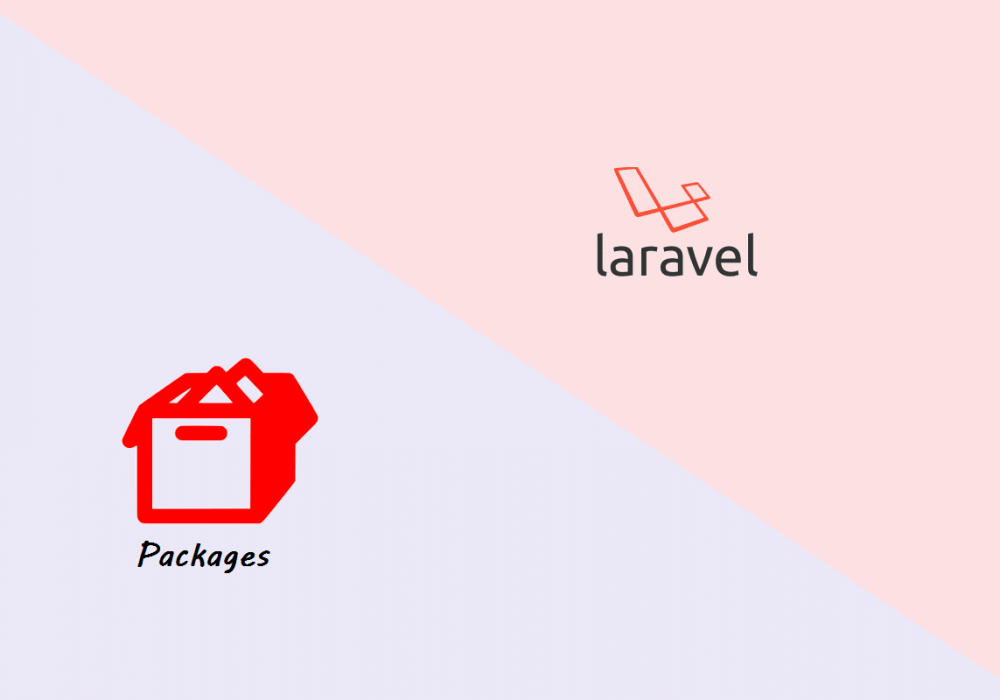
Simple Steps to Create Your First Laravel Package
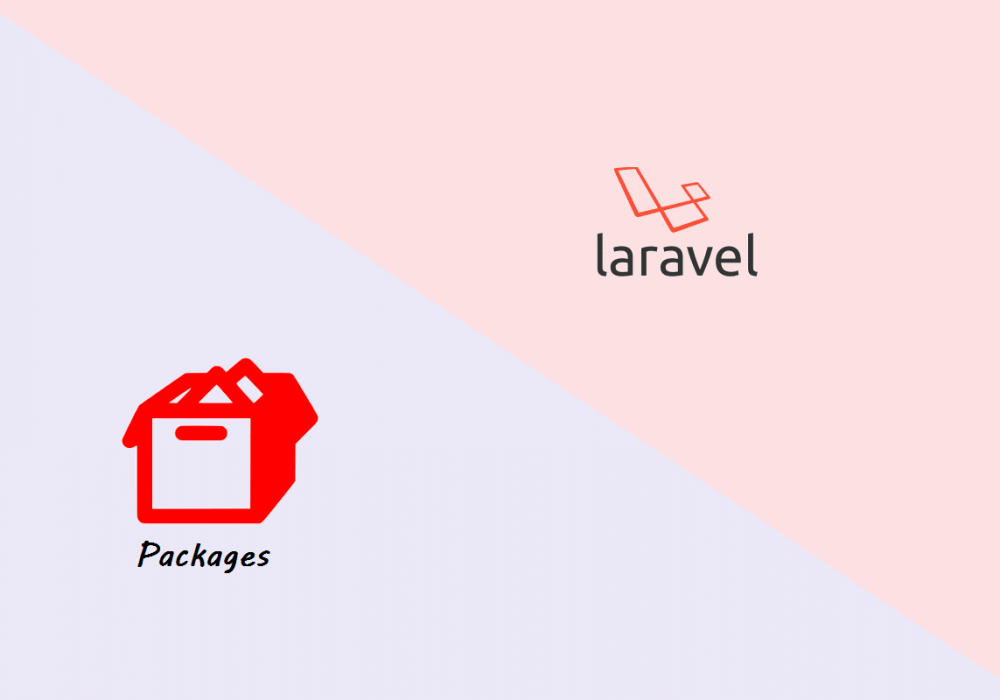
Packages are significantly important in adding functionality to your laravel project. As you may already see Carbon is also a package to handle date operation in laravel. Packages may vary based on unique functionalities that developers wanted to give benefit to their users. These packages may have views, controllers, routes, and some specific configuration to add on and enhance the application. You may sense that you might need a package or create one when you encounter a scenario where you repeatedly use or reuse certain features across multiple applications. In some cases, you want to give out your solution technique or research to other developers when they face the same problems and wanted to solve with your solution.
There are few reasons why we develop a package
- Reusability: this means that we can use a package again and again across multiple laravel projects if we need the specific functionalities implemented in that package. And so either other developers can also use if we deploy our package as a public.
- Expandability: we can customize your views, controllers, and models beyond what basic functionality laravel has and reuse them all over again.
- Ease of Integration: with a single command "composer require" we can bring it to the project and immediately take advantage of it.
- Complete disconnect from a specific project's domain/logic
In this blog post, you will learn very simple steps to create the first-ever laravel package and then upload it for public usage in the PHP package manager. In the below example we will create a package where its functionality is to get the number and translate or convert it into Khmer Words. We already published it for users to use in this link and we want to structurize this into a package for other developers to use as well.
Create Package
- Make sure we have composer installed on your computer
composer --version
- Create a folder to store package project and run
composer init
to create filecomposer.json
- Create a folder "src" to store source code, we need to tell Composer to map the package's namespace to that specific directory when creating the autoloader. We can register our namespace under the "psr-4" autoload key in the
composer.json
file as follows
{
"name": "sethathay/number-to-khmer-words",
"description": "A laravel package to convert number to khmer words",
"license": "MIT",
"authors": [
{
"name": "SETHA THAY",
"email": "sethathay@gmail.com"
}
],
"require": {},
"autoload": {
"psr-4": {
"SethaThay\\NumberToKhmerWords\\": "src"
}
}
}
- Create Your Own Service Provider that extends
Illuminate\Support\ServiceProvider
and implements aregister()
and aboot()
method. We have to create in folder src
<?php
namespace SethaThay\NumberToKhmerWords;
use Illuminate\Support\ServiceProvider;
class NumberToKhmerWordsServiceProvider extends ServiceProvider
{
public function register()
{
//
}
public function boot()
{
//
}
}
- To automatically register it with a Laravel project using Laravel's package auto-discovery we add our service provider to the "extra"> "laravel"> "providers" key in our package's
composer.json
{
...,
"autoload": { ... },
"extra": {
"laravel": {
"providers": [
"SethaThay\\NumberToKhmerWords\\NumberToKhmerWordsServiceProvider"
]
}
}
}
- Create a facade component by creating a class to handle your business logic and then integrate with the facade in a new
src/Facades
folder and finally register into service provider
Write your business logic here...
Create new facade src/Facades/NumberToKhmerWords.php
<?php
namespace SethaThay\NumberToKhmerWords\Facades;
use Illuminate\Support\Facades\Facade;
class NumberToKhmerWords extends Facade
{
protected static function getFacadeAccessor()
{
return 'numbertokhmerwords';
}
}
Register to Service provider NumberToKhmerWordsServiceProvider.php
<?php
namespace SethaThay\NumberToKhmerWords;
use Illuminate\Support\ServiceProvider;
class NumberToKhmerWordsServiceProvider extends ServiceProvider
{
public function register()
{
//
$this->app->bind('numbertokhmerwords', function($app) {
return new NumberToKhmerWords();
});
}
public function boot()
{
//
}
}
Now you have just created a new package using a skeleton Service Provider and Facade.
Using Package Locally
To help and test this newly created package, we can require this package in a local Laravel project. Follow the below steps to install the package into the local project development
- Create a new project
composer create-project laravel/laravel example-app
- Add the following "repositories" key below the "scripts" section in
composer.json
file of your Laravel app (replace the "url" with the directory where your package lives)
{
"scripts": { ... },
"repositories": [
{
"type": "path",
"url": "../../packages/number-to-khmer-words"
}
]
}
- You can now require your local package in the Laravel application using your chosen namespace of the package. Following our example, this would be
composer require sethathay/number-to-khmer-words
Deploy Package
Once we complete the package development, we can publish the package for public usage on the Packagist which is a default composer package repository that lets developers find packages and use them in project.
- First, let create a repository on Github and push the package to the repo
- Register a new account or login using your account with Packagist, then submit package by copy/paste the Github repository URL as below
- Once deployment process is finished, you will see the screen as below
- Next, we have to set up Github Hook so whenever we update code to the Github repository the package in the Packagist also updates as well. You can do that by getting an API token from your Packagist profile, then go to menu settings >> Webhooks and configure as below and then click the button create webhook
- Finally, you can create a release tag in Github and wait for a few seconds it will publish to the Packagist automatically
Using Package
You can use the published package just like other packages by using the composer require to include it into project.
composer require sethathay/number-to-khmer-words
You can use the package by facade and method which is defined in facade class. In our case
NumberToKhmerWords::show("00001200")
In conclusion, there are many ways and styles to define your own packages. In our showcase, we use the simplest one that utilizes only Service Provider and Facade to identify the input number and translate it to words (KHMER). You can customize your own packages in different ways with routes, controllers, views configuration, etc. You can follow this guideline from LaravelPackage.com for development which we found out super useful.
THANK YOU!!!